Abstract: 学习PHP的三个练习,第一个是判断一个数是否为素数,第二个是画五角星,第三个是写一个新闻界面,用读写文件来代替数据库。
题目一:判断一个数是否为素数
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| <html> <head> <meta charset="UTF-8"> <title> 判断一个数是否为素数 </title> </head> <body> <form action="judgePrime.php" method="get"> 请输入一个数:<input type="text" name="number"> <input type="submit" name="submit" value="提交"> </form> <?php $num = isset($_GET['number']) ? $_GET['number']: 2; $k = 0; for($i=1; $i<=$num; $i++){ if($num%$i == 0){ $k++; } } if($_GET['number']!=NULL) { if ($k == 2) { echo $num . '是一个素数'; } else { echo $num . '不是一个素数'; } } ?>
</body> </html>
|
OUT
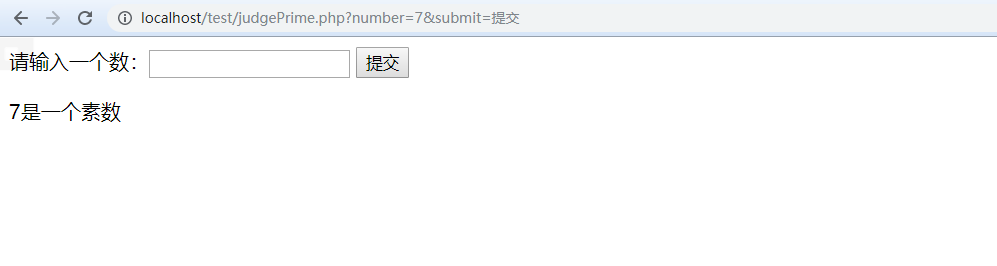
题目二:用PHP画五角星
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| <?php echo "\n\n"; for ( $i1 = 1; $i1 < 6; $i1++ ) /*这是五角星的上面一个角*/ { for ( $j1 = 1; $j1 < 19 - $i1; $j1++ ) printf( " " ); for ( $k1 = 1; $k1 <= 2 * $i1 - 1; $k1++ ) printf( "*" ); printf( "\n" ); }
for ($i2 = 1; $i2 < 5; $i2++ ) /*这是五角星的中间两个角*/ { for ( $j2 = 1; $j2 < 3 * $i2 - 3; $j2++ ) printf( " " ); for ( $k2 = 1; $k2 <= 42 - 6 * $i2; $k2++ ) printf( "*" ); printf( "\n" ); }
for ( $i3 = 1; $i3 < 3; $i3++ ) /*这是中间与下部相接的部分*/ { for ( $j3 = 1; $j3 < 12 - $i3; $j3++ ) printf( " " ); for ( $k3 = 1; $k3 <= 12 + 2 * $i3; $k3++ ) printf( "*" ); printf( "\n" ); }
for ( $i4 = 1; $i4 < 5; $i4++ ) /*这是五角星的下面两个角*/ { for ( $j4 = 1; $j4 < 10 - $i4; $j4++ ) printf( " " ); for ( $k4 = 1; $k4 <= 10 - 2 * $i4; $k4++ ) printf( "*" ); for ( $m4 = 1; $m4 < 6 * $i4 - 3; $m4++ ) printf( " " ); for ( $n4 = 1; $n4 <= 10 - 2 * $i4; $n4++ ) printf( "*" ); printf( "\n" ); }
?>
|
OUT
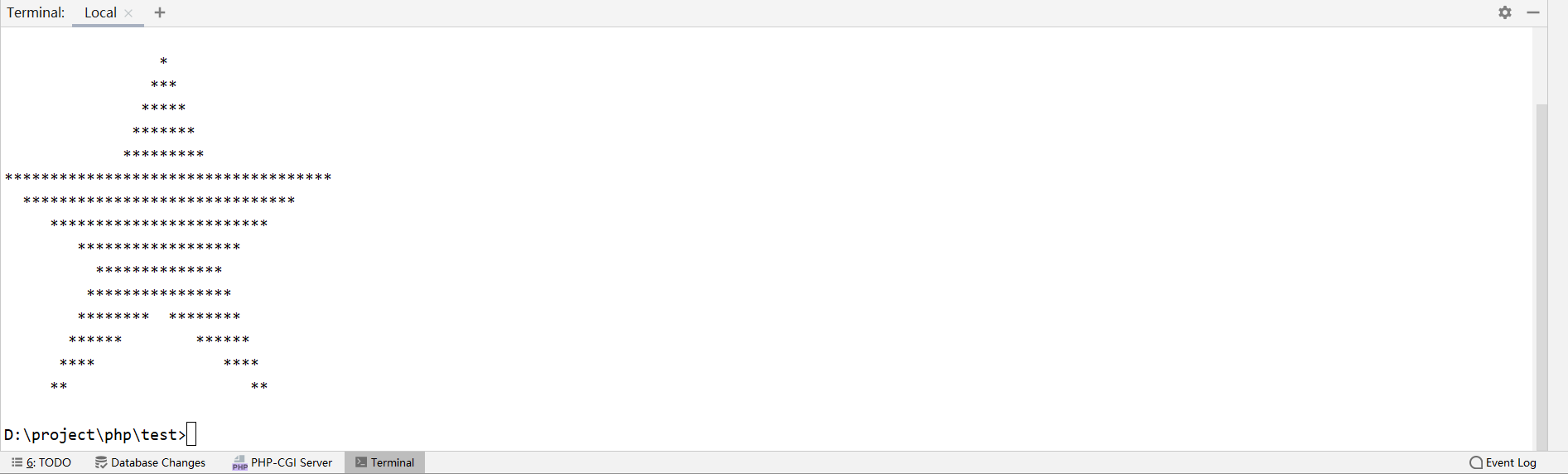
题目三:新闻界面
在news_file
文件夹下有index.php
和data
文件夹,index.php
主要是显示新闻的作用,data
文件夹里面有add_news.php
和news.db
,其中add_news.php
主要是添加新闻到news.db
中,news.db
每行存放着新闻的标号,标题,内容,日期,作者,热度和图片链接,其中标题,内容与作者使用了base64
编码,两两用||
分隔。
index.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57
| <html> <head> <meta charset="UTF-8"> <title>news</title> </head>
<body> <br>
<h1 align="center">ICQ新闻中心</h1> <hr> <table align="center"> <tr> <th>热度</th> <th>标题</th> <th>作者</th> <th>时间</th> <th>素质三联</th> </tr> <?php //用只读方式打开news.db文件 $fhandle = fopen("./data/news.db", "r"); //设置一个计数器,小于3时,每个文章标题下面增加图片和简介 $top3Cnt=1; while(!feof($fhandle)) { $fline = fgets($fhandle); $data = explode('||', $fline); $title = base64_decode($data[1]); $content = base64_decode($data[2]); $date = $data[3]; $author = base64_decode($data[4]); $hot = $data[5]; $imgUrl = $data[6]; echo <<<EOF <tr align="center"> <td>$hot</td> <td><a href="myl.php">$title</a></td> <td>$author</td> <td>$date</td> <td><button>👍</button>/<button>👎</button></td> </tr> EOF; if($top3Cnt<=3){ $tmpContent = mb_substr(base64_decode($data[2]), 0,50); echo <<<EOF <tr> <td><img src="$imgUrl" height="80" width="80"></td> <td colspan='4'><b>内容简介:</b>$tmpContent</td> </tr> EOF; } $top3Cnt++; } ?> </table> </body> </html>
|
OUT
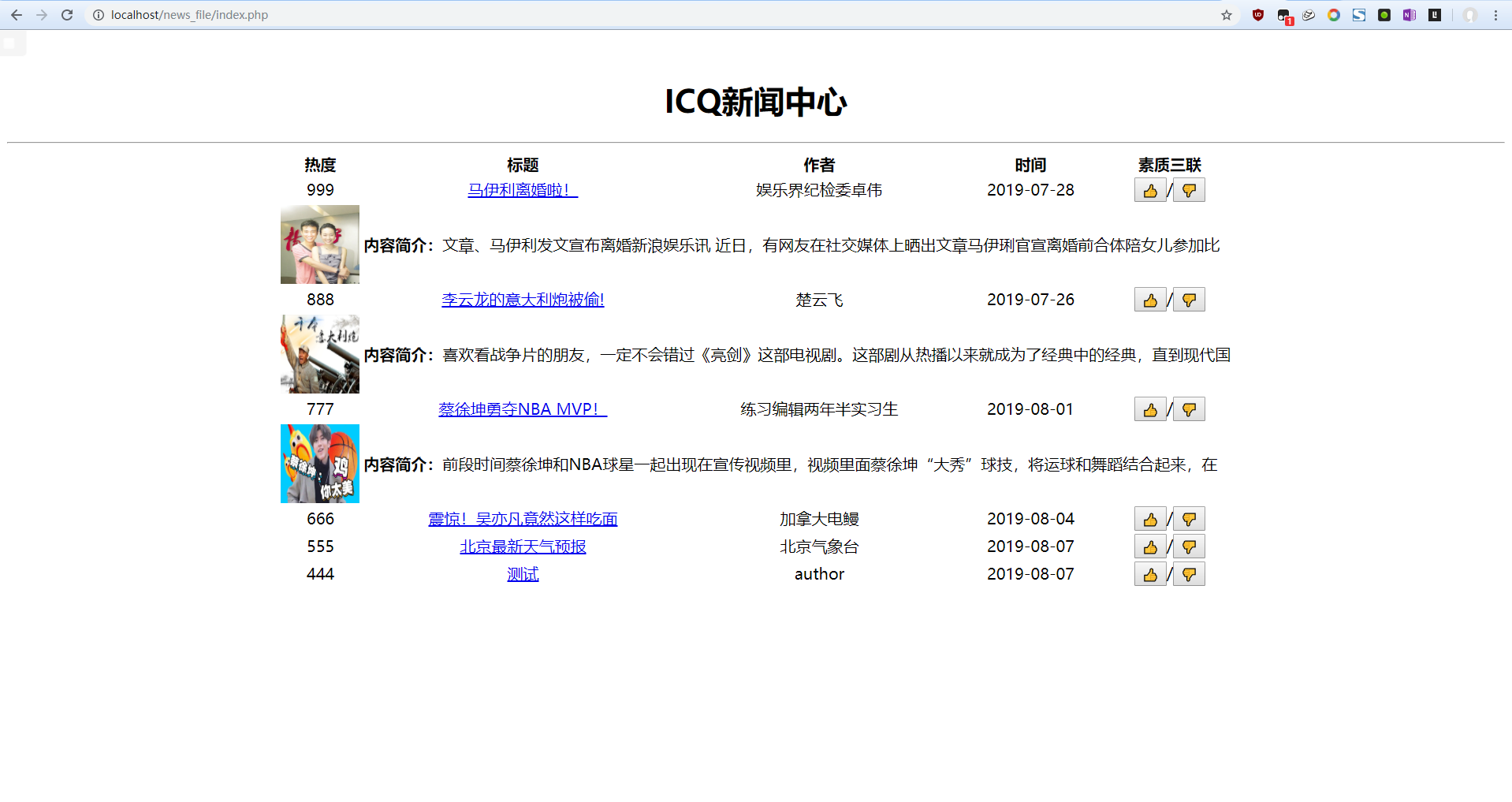
add_news.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <html> <head> <meta charset="UTF-8"> <title>增加新闻</title> </head>
<body> <form action="add_news.php" method="post"> 标号:<input type="number" name="id"><br> 标题:<input type="text" name="title"><br> <!-- 标题:<input type="text" namne="title"><br>--> 内容:<input type="text" name="content"><br> 日期:<input type="date" name="date"><br> 作者:<input type="author" name="author"><br> 热度:<input type="number" name="hot"><br> 图片:<input type="url" name="imgUrl"><br> <input type="submit" name="submit" value="提交"> </form>
<?php $fhandle = fopen('news.db ', 'a'); $arrMes = array(); @$arrMes[0] = $_POST['id']; @$arrMes[1] = base64_encode($_POST['title']); @$arrMes[2] = base64_encode($_POST['content']); @$arrMes[3] = $_POST['date']; @$arrMes[4] = base64_encode($_POST['author']); @$arrMes[5] = $_POST['hot']; @$arrMes[6]= $_POST['imgUrl']; if($arrMes[0]!=NULL) { $writeMes = implode('||', $arrMes); echo $writeMes; $fline = fputs($fhandle, $writeMes); } fclose($fhandle); ?>
</body> </html>
|
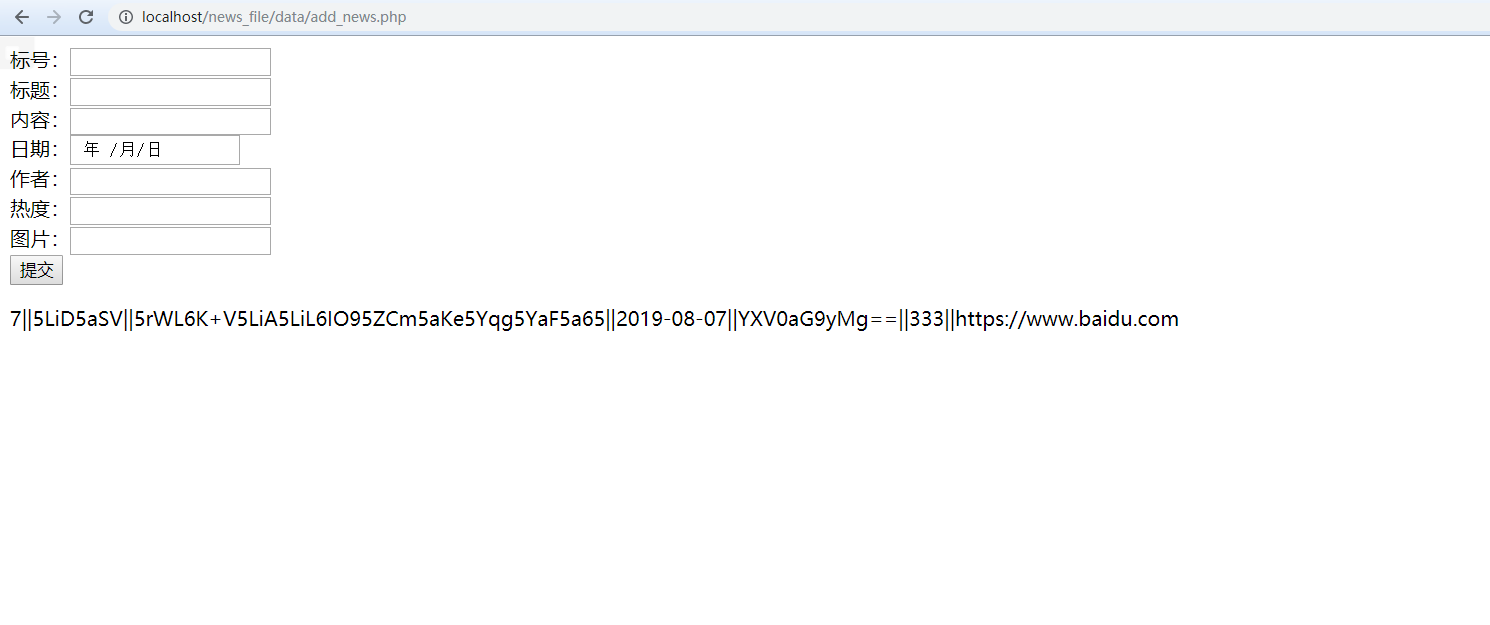